Everything
about Bitmaps
By:
Rohan Varma and Boice Wong
Introduction
This tutorial will teach you
about bitmaps(images). First it will teach you how to load a bitmap and draw it
on a screen. Then it will show you how to get that image to move around on the
screen. Next, it will illustrate how to get the image to appear wherever the
user touches the screen. Finally, the tutorial will demonstrate how to animate
a sprite. The different sections of the tutorial use the same code, so they
should all be done in the same project.
Drawing an Image on the Screen
- The first step is to find an image
that you want to display on the screen. Make sure that it is a PNG file or
it will not work. Put this picture into the drawable folder of your
project (it will be inside the res folder)
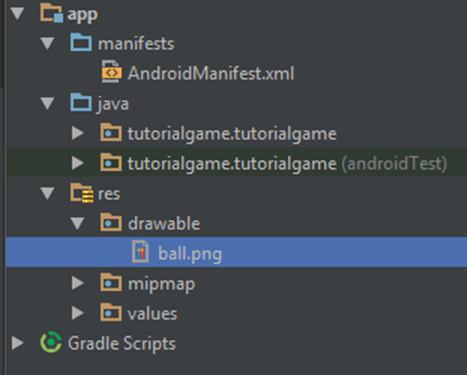
- In order to reference the image we
use the Bitmap class. However, the Bitmap is just the image, and in order
to specify where the image is drawn we use the Rect class. Create a global
Bitmap and Rect object.
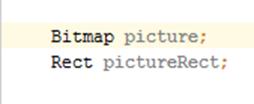
- The next
thing to do is to link the Bitmap to the image in the drawable folder. To
do this, add the following lines of code in the initialize method.
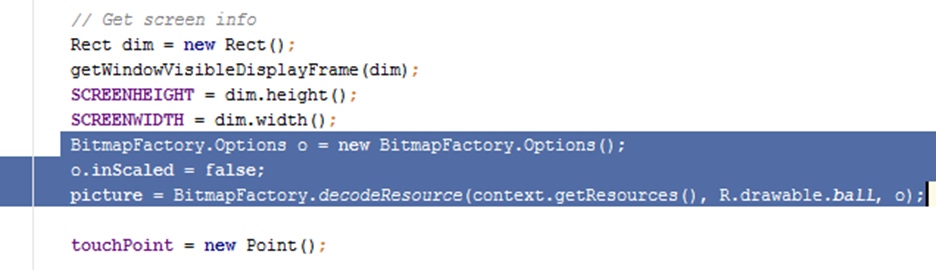
- Now that the
bitmap is initialized, we have to specify where we want to draw it. We do
this by setting the coordinates of the pictureRect we created. The
coordinate system of the screen is slightly different than one would
expect, with the origin being at the top left corner of the screen
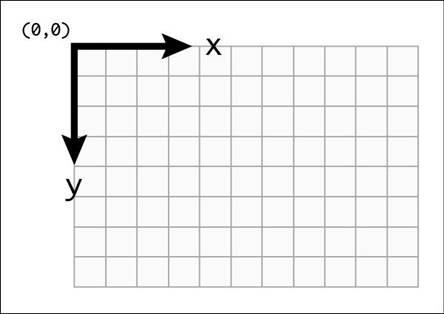
In
order to set up the Rect, add the following line of code. The 4 values in the
parentheses are the coordinates for where the left, top, right, and bottom of
the rectangle will be on the screen.

- The last thing to do now is to get
the program to draw the picture on the screen. This is done in the onDraw
method. In order to draw the image on the screen add the following line of
code in the onDraw method.
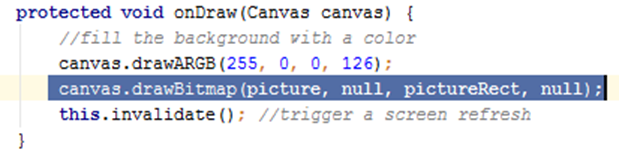
Now,
when you run the app, it will draw an image on the screen. When making a game
you could potentially reset the Rects coordinates in the Update method and have
images move around! If you want to learn how to do that, keep scrolling down.
Making a Bitmap Move
- Making a Bitmap move is a fairly
simple process. This section will show you how to get a Bitmap to move
around the screen and also how to prevent the bitmap from moving off the
screen. All this will be done in the Update method. Note that Update and
onDraw are asynchronous (meaning that onDraw isn’t always called after
Update has run), so it is important to make sure that onDraw is only used
to draw things on the screen and doesn’t actually change any of the rects
coordinates.
- The first thing to do is to
initialize all the global variables we will need. Make ints for xSpeed,
ySpeed, xPosition, and yPosition. Also, for convenience create ints for
pictureWidth and pictureHeight.

- Now in Initialize(), set xPos equal
to the left coordinate of pictureRect, and yPos equal to the top
coordinate of pictureRect. Set xSpeed and ySpeed equal to 1 and
pictureWidth and pictureHeight equal to picture.getWidth() and
picture.getHeight() respectively. You have now initialized all the
variables you will need.
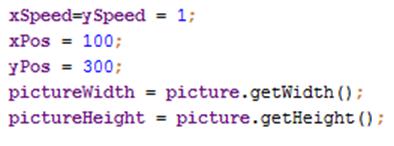
- The next thing to do is to change
pictureRect’s position in Update(). Scroll down to the update method and
add xSpeed to xPos and ySpeed to yPos. Then, change pictureRects position
by using the Rect.set() method to (xPos,yPos,xPos+pictureWidth,yPos+pictureHeight).

Make
sure you put this in the Update() method !!!!! If
you run the program at this point, you should have an image that moves off the
screen. If the image is too slow or fast, you can either adjust xSpeed or
ySpeed, or change the long in the try catch statement in update
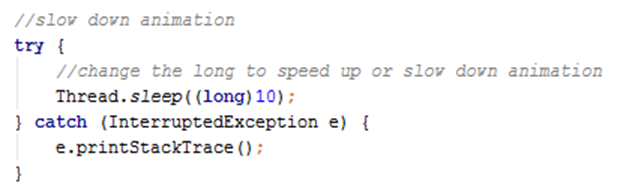
- The image now moves, but it goes off
the screen. In order to do this we have to be able to detect when the
image is about to move off the screen and multiply either the xSpeed or
ySpeed by negative 1. In order to do this we compare the xPos and yPos to
the height and width of the screen. If you look at the top of initialize,
you’ll notice that the game template includes code that finds the height
and width of the screen and puts it into two variables, SCREENHEIGHT and
SCREENWIDTH respectively. Using these ints, we can detect when the image
is going to go off the screen and appropriately reverse the speed. To do
this, put the following code into Update(), before the pictureRect.set()
line
.
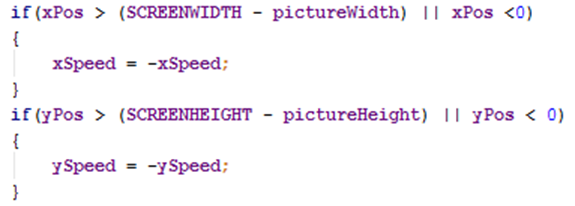
- Now, when you
run the program, the image should no longer go off the screen!!
Getting the Image to Appear
Where the User Touches the Screen
- This is also
a fairly simple process, as the game template has almost everything you
need. All you need to do is put this if statement into Update before the
pictureRect.set() line.
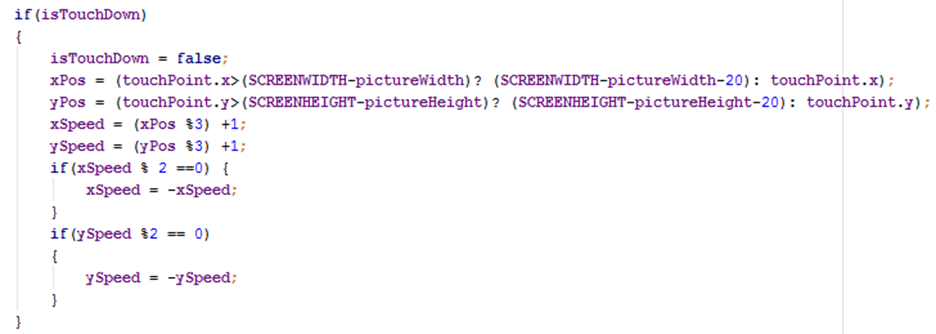
Animating a Sprite
- Animating a sprite is similar to
putting an image on the screen. Again, the first step is to find an image
that you want to use. The difference however is that the image has to be a
strip of images with the animations desired. Put this sprite strip in the
drawable folder as well.

- A few necessary characteristics of
the sprite strip is that it must be transparent and the images need to be
evenly spaced apart. Images can be made transparent using programs such as
photoshop and gimp
- Just like the images, you need to
initialize the sprite strip in the same way.
- Now the program needs to decide to
show only the individual sprites and not the whole strip. Do this by
creating a new int and giving it a value of 0. Then initialize your int
and set the value equal to the number of animations. In the case of the
sprite strip shown the int number would be 7.
- Just as
before we need to set up a rectangle for the image. We also need to set up
a rectangle for the sub sprites. Also create new ints for the Width and
Height values.
The 4 values in parentheses are the same as before; left, top,
right, bottom.
- Now to alternate between the sub
sprites, you need this if statement in the Update method.

- If you need to slow down the speed of
the animation, you can use this code to do so
This basically makes a period of time in which
the program will wait to change sub sprites.
- The last step is to draw it on the
screen. go to the onDraw method like before and use this code to place it
on the screen.
It is
the same as the code we used before except in canvas.drawBitmap, the
second value will be your sub sprite rectangle.
- Now, when you
run the app, it will draw an animated image on the screen. Explore using
different orders of the sprite strip and have fun creating your own!